GUI Programming:
Pythons Tutorials
GUIs provide a visually appealing and interactive way for users to interact with your programs, making your applications more user-friendly and engaging.
GUI programming involves creating windows, buttons, text boxes, and other graphical elements that users can interact with, making your programs more intuitive and engaging.
1. Choosing a GUI Library:
Python offers various GUI libraries, such as Tkinter, PyQt, and wxPython, that allow you to create GUI applications. In this tutorial, we’ll focus on Tkinter, which comes bundled with Python.
2. Setting Up Tkinter:
To use Tkinter, you need to import it and create a main application window.
Example: Setting Up a Tkinter Window
import tkinter as tk
root = tk.Tk()
root.title("My GUI Application")
3. Setting the Window Size:
You can set the size of the window using the geometry()
method, which takes a string argument in the format “widthxheight.”
Example: Setting the Window Size
window_width = 800
window_height = 600
root.geometry(f"{window_width}x{window_height}")
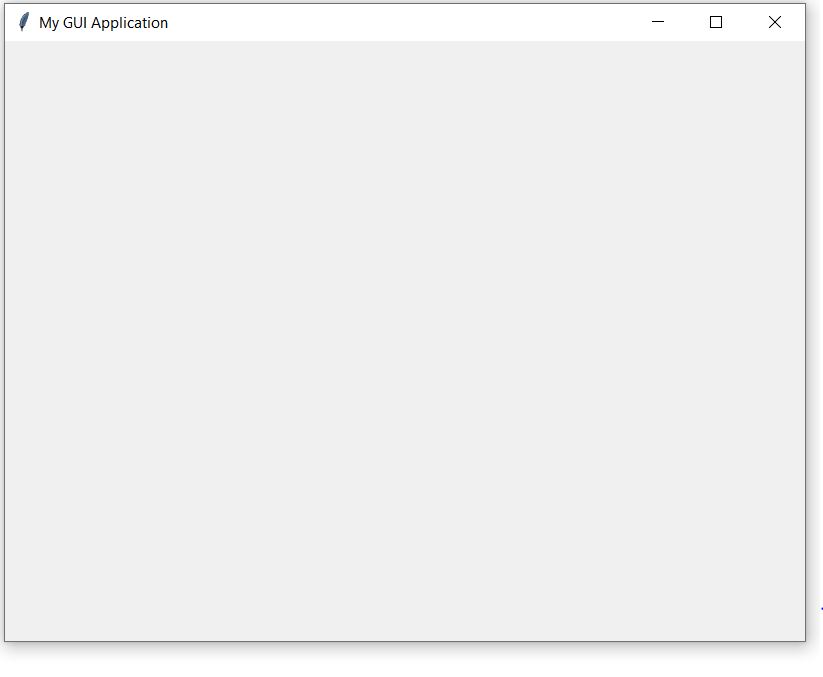
4. Adding Widgets:
Widgets are the building blocks of a GUI, including buttons, labels, text boxes, and more. You can add widgets to your main window to create the interface.
Example: Adding Widgets
label = tk.Label(root, text="Hello, GUI!")
button = tk.Button(root, text="Click me")
label.pack()
button.pack()
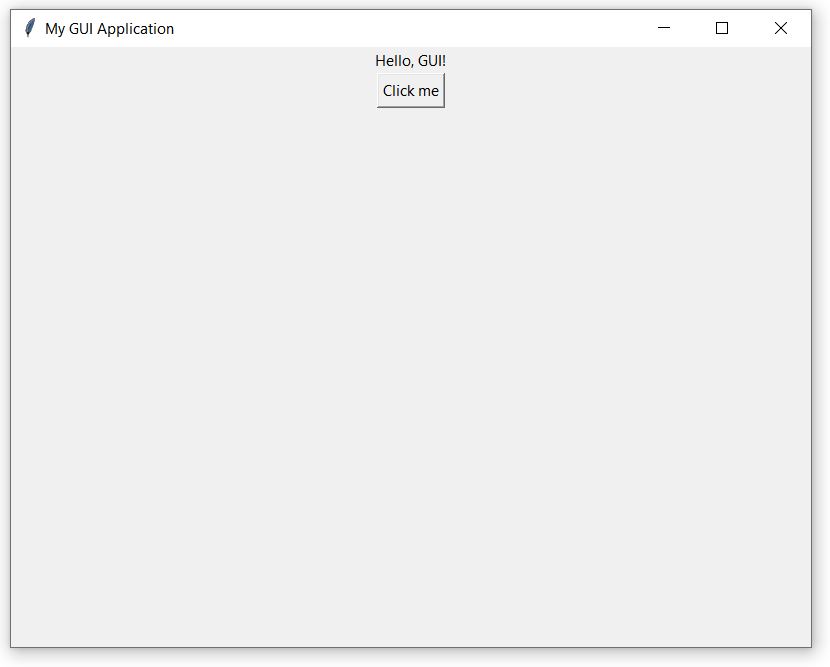
5. Managing Layout:
Tkinter offers various layout managers like pack()
, grid()
, and place()
to arrange widgets within your window.
Example: Using the grid() Layout Manager
label1 = tk.Label(root, text="Label 1")
label2 = tk.Label(root, text="Label 2")
label1.grid(row=0, column=0)
label2.grid(row=1, column=0)
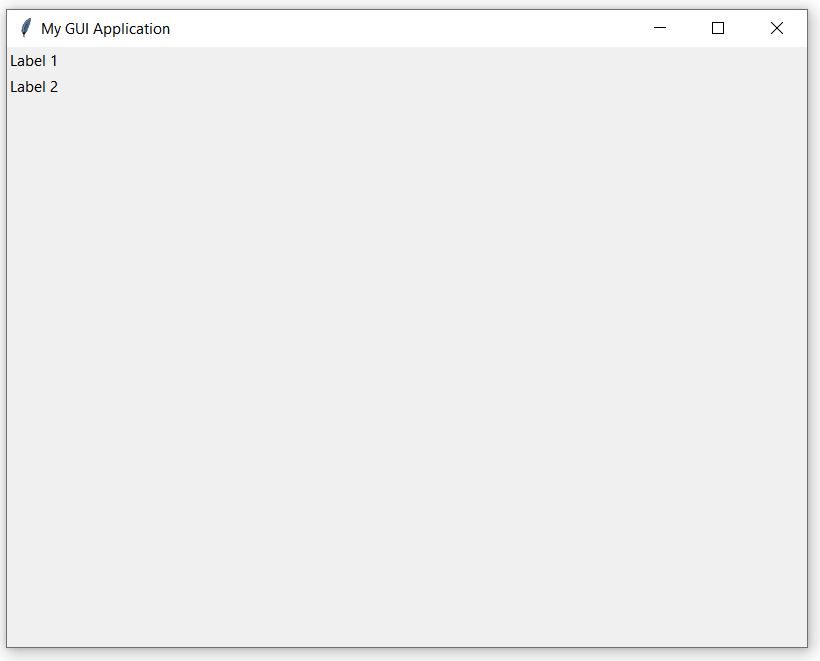
6. Handling Events: Responding to User Actions Widgets can trigger events like button clicks. You can bind functions to these events to define how your program responds.
Example: Handling Button Clicks
def button_click():
label.config(text="Button clicked!")
button = tk.Button(root, text="Click me", command=button_click)
button.pack()
label = tk.Label(root, text="")
label.pack()
6. Creating More Complex Interfaces:
You can create more complex interfaces by combining different widgets and layout managers, allowing users to interact with your program in various ways.