Learn How To Create Realistic Lightning Effects With Bezier Curves and Noise in JavaScript
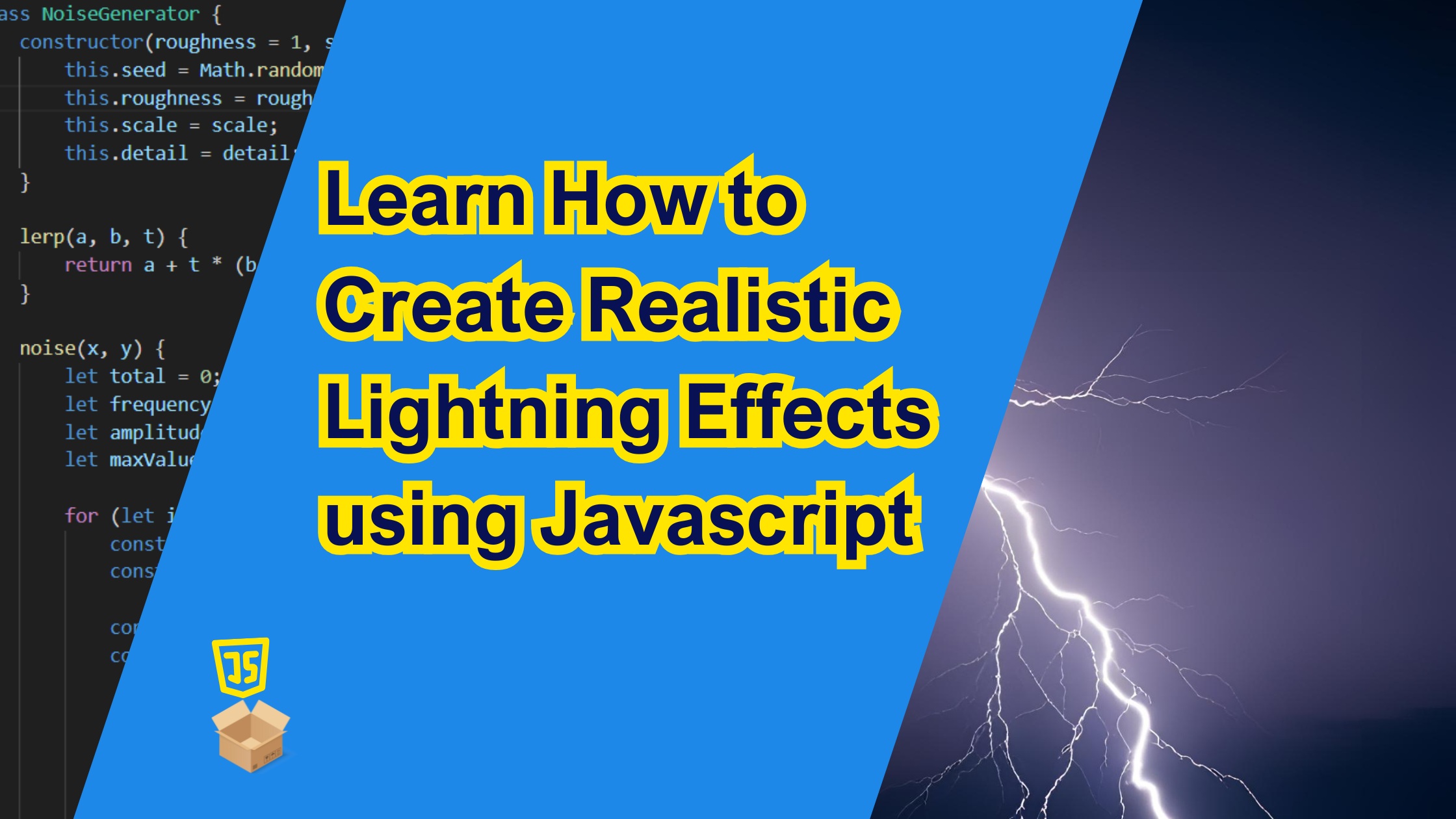
Ever wanted to create jaw-dropping lightning effects with JavaScript? You are in the right place! In this tutorial, we are going to explore how to use Bezier curves, noise functions, and dynamic branching to bring realistic lightning to life on the HTML5 Canvas. Let’s dive in!
Prerequisites
Before we get started, make sure you have a basic understanding of HTML, CSS, and JavaScript.
- Basic Understanding of HTML, CSS, and JavaScript: Familiarity with the fundamental concepts of HTML for structuring web pages, CSS for styling, and JavaScript for adding interactivity is essential. This tutorial assumes a basic knowledge of these languages.
- Web Browser: Use a modern web browser like Google Chrome, Mozilla Firefox, or Microsoft Edge.
For the purpose of this tutorial, you can download the source files for the demo to help you follow along:
Note: As you test the source files, ensure that you load them within a suitable environment such that all the files are loaded. For offline use, we recommend that you use Live Server and vscode to load the files. Simply open the files in Vscode and run “index.html” using Live Server.
Demo
Here is what we are going to create.
Understanding the Basics
To achieve the lightning effect, we use three main components; vector points and math, bezier curves and a noise generator. In summary, our code is designed to dynamically generate and animate lightning using the following elements:
- Vectors helps to deal with necessary mathematics.
- Bezier curves to shape the lightning.
- A noise generator for realistic jagged edges.
Setting Up the Project
Let's start by setting up the basic HTML structure for our project. Create an HTML called "index.html", with a basic HTML web format, and add a canvas element.
Creating the Canvas Element
The HTML structure consists of a <canvas>
element, where the animation is rendered, and a set of range sliders that allow users to control parameters like roughness, scale, detail, and lightning branch properties.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
</head>
<body>
<!-- Canvas element to render the effect -->
<canvas id="canvas" width="500px" height="500px" style="background: black;"></canvas>
<!-- UI controls for adjusting noise and lightning settings -->
<span style="display:flex;flex-direction: row;gap:20px;font-family:sans-serif;">
<div>
<label for="roughness" style="font-weight: bold;">Noise:</label><br>
<label for="roughness">Roughness:</label>
<input type="range" id="roughness" min="0.1" max="1" step="0.1" value="1">
<br>
<label for="scale">Scale:</label>
<input type="range" id="scale" min="0" max="5" step="0.1" value="3">
<br>
<label for="detail">Detail:</label>
<input type="range" id="detail" min="0" max="10" value="10">
</div>
<div>
<label for="roughness" style="font-weight: bold;">Lightning:</label><br>
<label for="branches">Max Branches:</label>
<input type="range" id="branches" min="0" max="100" step="1" value="3">
<br>
<label for="strength">Max Branch Threshold:</label>
<input type="range" id="strength" min="0" max="10" step="1" value="3">
<br>
<label for="spacing">Branch Spacing</label>
<input type="range" id="spacing" min="0" max="100" step="0.01" value="80">
</div>
</span>
</body>
</html>
Lets also add script tag just below the <span> section as shown below. Inside this tag, we shall add our JavaScript code.
<script type=text/javascript>
</script>
Lets Dive Into the Code
The JavaScript code is where the magic happens. Here’s a breakdown of its core components:
1.Setting Up the Canvas
This initializes the canvas and sets up the drawing context.
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const width = canvas.width;
const height = canvas.height;
// Frame rate settings
const timeout = 1000 / 60; // 60 FPS
2. Simulation Settings
To help control the settings of our simulation, we will setup some variables using a settings object. These settings will be controlled by the input controls.
// Settings for tree generation
const settings = {
branch_threshold: 2, // Minimum branching strength
max_branches: 10, // Maximum branches allowed
branch_spacing: 100, // Space between branches
detail: 10, // Level of detail
scale: 3, // Scale factor for noise generation
roughness: 1, // Roughness factor for noise
// Load settings from HTML input elements
load: function () {
this.max_branches = parseInt(this.get_setting("branches"));
this.branch_threshold = parseInt(this.get_setting("strength"));
this.branch_spacing = parseFloat(this.get_setting("spacing"));
this.detail = parseInt(this.get_setting("detail"));
this.scale = parseInt(this.get_setting("scale"));
this.roughness = parseFloat(this.get_setting("roughness"));
},
// Get values from input elements by their ID
get_setting: function (setting) {
var element = document.getElementById(setting);
return element.value;
}
}
3. Vector Class for Geometry Calculations
We define a vec2d
class to handle 2D vector operations such as midpoint calculations, normal vectors, and scaling:
// Vector class for 2D points
class vec2d {
constructor(x, y, length = 0, tangent = null, normal_1 = null, normal_2 = null) {
this.x = x;
this.y = y;
this.spline_length = length; // Length of the spline segment
this.normal_1 = normal_1;
this.normal_2 = normal_2;
this.tangent = tangent;
this.thickness = 0; // Line thickness
}
// Calculate the normal vector between two points
get_normal(p1, p2) {
let dx = p2.x - p1.x;
let dy = p2.y - p1.y;
let length = Math.sqrt(dx * dx + dy * dy);
if (length === 0) return null; // Avoid division by zero
return new vec2d(-dy / length, dx / length);
}
// Copy a vector
copy() {
return new vec2d(this.x, this.y, this.spline_length);
}
// Add two vectors
add(p1, p2) {
return new vec2d(p1.x + p2.x, p1.y + p2.y);
}
// Subtract two vectors
subtract(p1, p2) {
return new vec2d(p2.x - p1.x, p2.y - p1.y);
}
// Scale a vector by a factor
scale(x) {
this.x *= x;
this.y *= x;
return this;
}
// Get the magnitude (length) of a vector
get_magnitude() {
return Math.sqrt(this.x * this.x + this.y * this.y);
}
// Compute the midpoint between two points
midpoint(p1, p2) {
return new vec2d((p1.x + p2.x) / 2, (p1.y + p2.y) / 2);
}
}
3. Helper functions
We are also going to create some helper functions to help us draw the lines and points for the lightning effect.
// Function to draw a point (circle) on the canvas
function point(vector, radius = 0, color = 'white') {
ctx.beginPath(); // Begin drawing a new shape
ctx.fillStyle = color; // Set the fill color
ctx.arc(vector.x, vector.y, radius, 0, Math.PI * 2, false); // Draw a full circle
ctx.fill(); // Fill the circle with the specified color
ctx.closePath(); // Close the path
}
// Function to draw a smooth curved line between two points
function line(p1, p2, thickness) {
const mid = p1.midpoint(p1, p2); // Compute the midpoint for smooth curvature
ctx.beginPath(); // Start a new path
ctx.lineJoin = "round"; // Ensure smooth joint connections
// Glow effect for the line
ctx.shadowBlur = 12; // Set glow intensity
ctx.shadowColor = 'rgba(173, 216, 230, 0.8)'; // Light blue glow color
ctx.strokeStyle = 'rgba(190, 120, 255, 1)'; // Line color (purple shade)
ctx.lineWidth = thickness; // Set line thickness
// Draw a quadratic curve from p1 to p2 using the computed midpoint
ctx.moveTo(p1.x, p1.y); // Start at p1
ctx.quadraticCurveTo(mid.x, mid.y, p2.x, p2.y); // Create a smooth curve through the midpoint
ctx.stroke(); // Render the stroke on the canvas
ctx.closePath(); // End the path
}
4. Noise Generator for Realistic Jagged Edges
The noise generator is created through the noise generator class which is used to generate random values to offset the points on our bezier curves in order to form the lightning zigzag shape.
class NoiseGenerator {
// Constructor initializes the noise generator with customizable parameters
constructor(roughness = 1, scale = 0.05, detail = 1) {
this.seed = Math.random() * 1000; // Random seed to introduce variation
this.roughness = roughness; // Controls the intensity of variations
this.scale = scale; // Determines the scale of noise features
this.detail = detail; // Number of octaves for noise (higher = more detail)
}
// Linear interpolation function
lerp(a, b, t) {
return a + t * (b - a);
}
// Generates Perlin-style noise based on x and y coordinates
noise(x, y) {
let total = 0; // Accumulated noise value
let frequency = this.scale; // Starting frequency
let amplitude = this.roughness; // Initial amplitude
let maxValue = 0; // Used to normalize the noise output
// Loop over multiple octaves to add more noise detail
for (let i = 0; i < this.detail; i++) {
// Convert coordinates into integer grid points
const X = Math.floor(x * frequency) & 255;
const Y = Math.floor(y * frequency) & 255;
// Compute fractional parts of x and y within the grid
const fx = x * frequency - Math.floor(x * frequency);
const fy = y * frequency - Math.floor(y * frequency);
// Smooth interpolation function (fade function)
const u = fx * fx * (3 - 2 * fx);
const v = fy * fy * (3 - 2 * fy);
// Generate pseudo-random gradient values based on hashed coordinates
const a = Math.sin((X + Y * 57 + this.seed) * 0.1);
const b = Math.sin((X + 1 + Y * 57 + this.seed) * 0.1);
const c = Math.sin((X + (Y + 1) * 57 + this.seed) * 0.1);
const d = Math.sin((X + 1 + (Y + 1) * 57 + this.seed) * 0.1);
// Bilinear interpolation of the four surrounding noise values
total += this.lerp(
this.lerp(a, b, u),
this.lerp(c, d, u),
v
) * amplitude;
// Normalize the output by keeping track of max amplitude
maxValue += amplitude;
amplitude *= this.roughness; // Reduce amplitude for next octave
frequency *= 2; // Increase frequency for finer details
}
// Normalize the result to keep it within a reasonable range
return total / maxValue;
}
}
5. The Bezier Curves
The BezierCurve
class is responsible for defining and drawing curved lightning paths. The BezierCurve object will be used to make an instance object of a lightning branch. In principle, the bezier curve is determined by three points; p0,p1 and p2. Using these points, we compute all the other points and put them along the bezier curve based on segment length. For each point we generate, we also offset it using the noise value from the noise generator to make the zigzag coordinates. We also generate and store the line or curve segments to be used to draw the lightning. In order to compute the direction and behaviour of the lines, we store special data on the vector points such as the tangent, and normals at specific points.
class BezierCurve {
// Constructor initializes a quadratic Bézier curve with control points
constructor(p0, p1, p2, thickness = 5, is_branch) {
this.p0 = p0; // Start point
this.p1 = p1; // Control point (influences curvature)
this.p2 = p2; // End point
this.is_branch = is_branch; // Indicates if this curve is a branch
this.thickness = thickness; // Defines the thickness of the curve
this.curvePoints = []; // Stores points along the curve
this.segmentLines = []; // Stores segment lines of the curve
}
// Calculates a point on the quadratic Bézier curve at parameter t
getPoint(t, length) {
const x = (1 - t) * (1 - t) * this.p0.x + 2 * (1 - t) * t * this.p1.x + t * t * this.p2.x;
const y = (1 - t) * (1 - t) * this.p0.y + 2 * (1 - t) * t * this.p1.y + t * t * this.p2.y;
if (t === 0) {
return this.p0.copy(); // Return start point if t = 0
} else if (t === 1) {
return this.p2.copy(); // Return end point if t = 1
}
return this.getOffset(new vec2d(x, y, length)); // Apply noise offset
}
// Computes the tangent (direction) of the curve at a given t value
getTangent(t) {
let dx = 2 * (1 - t) * (this.p1.x - this.p0.x) + 2 * t * (this.p2.x - this.p1.x);
let dy = 2 * (1 - t) * (this.p1.y - this.p0.y) + 2 * t * (this.p2.y - this.p1.y);
let magnitude = Math.sqrt(dx * dx + dy * dy);
return magnitude === 0 ? new vec2d(0, 0) : new vec2d(dx / magnitude, dy / magnitude); // Normalize vector
}
// Adds a noise-based offset to the given point for a natural, organic look
getOffset(point) {
const noiseGen = new NoiseGenerator(settings.roughness, settings.scale, settings.detail);
const noiseX = noiseGen.noise(point.x * 0.05, point.y * 0.05) * 10;
const noiseY = noiseGen.noise(point.y * 0.05, point.x * 0.05) * 10;
return new vec2d(point.x + noiseX, point.y + noiseY, point.spline_length);
}
// Computes the Euclidean distance between two points
distance(p1, p2) {
return Math.sqrt((p2.x - p1.x) ** 2 + (p2.y - p1.y) ** 2);
}
// Generates points along the Bézier curve with specified segment length
generatePoints(segmentLength = 5) {
this.curvePoints = [];
this.segmentLines = [];
let prevPoint = this.p0;
let prevSegment = null;
let newSegment = null;
this.accumulatedLength = 0;
this.curvePoints.push(prevPoint);
// Iterate over the curve in small steps
for (let t = 0.1; t <= 1; t += 0.02) {
const newPoint = this.getPoint(t, this.accumulatedLength);
newPoint.tangent = this.getTangent(t); // Compute tangent
newPoint.thickness = this.thickness; // Assign thickness
if (prevSegment !== null) {
newPoint.normal_1 = newPoint.get_normal(prevSegment.start, prevSegment.end);
}
this.accumulatedLength += this.distance(prevPoint, newPoint);
// Add point to curve only if segment length is met
if (this.distance(prevPoint, newPoint) >= segmentLength) {
this.curvePoints.push(newPoint);
newSegment = { start: prevPoint, end: newPoint };
newPoint.normal_2 = newPoint.get_normal(newSegment.start, newSegment.end);
this.segmentLines.push(newSegment);
prevPoint = newPoint;
prevSegment = newSegment;
}
}
}
// Draws the Bézier curve with varying thickness
draw() {
let maxThickness = this.thickness;
let minThickness = 0;
let totalLength = this.accumulatedLength;
let index = 0;
// Iterate over curve segments and render them
this.segmentLines.forEach(({ start, end }) => {
let thickness = maxThickness;
if (index === 0) {
end.thickness = thickness; // Maintain max thickness at start
} else {
let progress = start.spline_length / totalLength;
thickness = maxThickness * (1 - progress) + minThickness * progress; // Gradually reduce thickness
end.thickness = thickness;
}
line(start, end, thickness); // Render segment with computed thickness
index += 1;
});
}
}
6. Creating Branches for Realistic Forking
In order to create the lightning forking, we use the BeizerInstance
class. This class helps to manage the number of branches as well as draw each branch.
class BeizerInstance {
// Constructor initializes a Bézier curve instance with branching capabilities
constructor(p0, p1, p2, thickness = 5, branch_strength = 0, segmentLength = 10, is_branch = false) {
// Create the main Bézier curve
this.bezierCurve = new BezierCurve(p0, p1, p2, thickness, is_branch);
// Segment length for curve discretization
this.segmentLength = segmentLength;
this.bezierCurve.generatePoints(segmentLength); // Generate points along the curve
// Base point of the instance (starting point)
this.base = p0;
// Strength of the branch (determines branching depth)
this.branch_strength = branch_strength;
// Holds generated branches
this.branches = [];
// Generate branches from the main curve
this.create_branches();
}
// Creates branches along the curve
create_branches() {
var main_branch = this;
// Check if the branch_strength is within the branching threshold
if (main_branch.branch_strength <= settings.branch_threshold) {
var curve = main_branch.bezierCurve;
var prev_p1 = null; // Stores the previous branching point
for (let i = 0; i < curve.curvePoints.length; i++) {
if (main_branch.branches.length < settings.max_branches) {
var p1 = curve.curvePoints[i];
// Ensure proper spacing between branches
if (prev_p1 !== null) {
if (p1.spline_length - prev_p1.spline_length <= settings.branch_spacing) {
continue;
}
}
// Prevent excessive branches
if (main_branch.branch_count > settings.max_branches) {
break;
}
if (p1 && p1.spline_length > 5) {
var normals = [];
// Collect available normals at the point
if (p1.normal_1 !== null) {
normals.push(p1.normal_1);
}
if (p1.normal_2 !== null) {
normals.push(p1.normal_2);
}
if (normals.length > 0) {
// Select a random normal for branching
var a = Math.floor(Math.random() * (normals.length - 1));
var normal = normals[a];
// Copy the tangent direction at this point
var tangent = p1.tangent.copy();
var n = Math.random();
// Define a random length for the new branch
var curve_length = 0.5 * Math.random() * (curve.accumulatedLength -p1.spline_length);
var dir;
if (tangent !== null && n > 0.5) {
// Slightly modify the direction using the normal
var alpha = Math.random() * 0;
var t = tangent;
dir = t.add(normal.scale(0.1), t).scale(curve_length);
} else {
dir = tangent.scale(100); // Default direction if no modification is applied
}
// Random factor to control the branching angle
var f = Math.random() * 2 + -1;
// Compute new branch points
var p2 = p1.add(p1, dir);
var p3 = p2.midpoint(p1, p2); // Midpoint between p1 and p2
// Compute normal at the midpoint
var normal_3 = p3.get_normal(p1, p2);
var offset = 80;
// Adjust the midpoint using the normal for a more organic look
p3 = p3.add(p3, normal_3.scale(offset * f));
// Increment branch strength to track branching depth
var strength = main_branch.branch_strength + 1;
// Create a new branch instance
var instance = new BeizerInstance(p1, p3, p2, p1.thickness, strength, 10, true);
main_branch.branches.push(instance);
}
}
}
prev_p1 = p1; // Update the previous branching point
}
}
}
// Draws the main curve and its branches
draw() {
this.bezierCurve.draw();
this.draw_branches();
}
// Regenerates the branches when needed
refresh_branches() {
this.bezierCurve.generatePoints();
this.branches = [];
this.branch_count = 0;
this.create_branches();
}
// Draws all branches recursively
draw_branches() {
this.branches.forEach(function (branch) {
branch.bezierCurve.thickness = branch.base.thickness; // Maintain thickness consistency
branch.draw(); // Recursively draw branches
});
}
}
7. Updating and Animating the Lightning Effect
Finally, we create a clear screen function to help us clear the screen after every frame. We initialize the main branch using a new BezierCurve Instance. We also add a mouse event to help us capture mouse coordinates and use them to set the end point of the Bezier curve. The compute_beizer_midpoint helps use to compute a smooth control point for the curve. We also create an update function which we call via the setTimeout function to start the animation loop.
// Function to clear the canvas with a semi-transparent black overlay
function clearScreen() {
ctx.globalCompositeOperation = "source-over"; // Reset blending mode to default
ctx.fillStyle = 'rgba(0,0,0,0.9)'; // Semi-transparent black for fading effect
ctx.fillRect(0, 0, width, height); // Fill the entire canvas
}
// Define three control points for the Bézier curve
const p0 = vec(250, 50); // Start point
const p1 = vec(500, 100); // Control point
const p2 = vec(250, 300); // End point
// Function to compute the midpoint control point for the Bézier curve
function compute_beizer_midpoint(p0, p2, p1) {
p1.x = (p0.x + p2.x) / 2 + (Math.random() * 20 - 10); // Add randomness to x-coordinate
p1.y = (p0.y + p2.y) / 2 - 50; // Raise the midpoint slightly
}
// Compute the initial position of p1
compute_beizer_midpoint(p0, p2, p1);
// Load settings for the Bézier tree generation
settings.load();
// Create a new Bézier instance with the computed control points
const instance = new BeizerInstance(p0, p1, p2, 4);
// Add an event listener to update the curve dynamically based on mouse movement
canvas.addEventListener("mousemove", (event) => {
const rect = canvas.getBoundingClientRect(); // Get canvas position relative to the window
// Update the endpoint (p2) to follow the mouse position
p2.x = event.clientX - rect.left;
p2.y = event.clientY - rect.top;
// Recalculate the midpoint control point dynamically
p1.x = (p0.x + p2.x) / 2 + (Math.random() * 20 - 10);
p1.y = (p0.y + p2.y) / 2 - 50;
});
// Define refresh intervals for branch regeneration
var initial_refresh = 10;
var refresh = initial_refresh;
// Main update function to animate the Bézier curve
function update() {
settings.load(); // Reload settings
clearScreen(); // Clear the screen before redrawing
// Refresh branches at defined intervals to create organic growth
if (refresh <= 0) {
instance.refresh_branches();
refresh = initial_refresh; // Reset refresh counter
} else {
refresh -= 1; // Decrease refresh timer
}
// Draw the initial starting point
point(p0, 2, "rgba(190, 190, 255, 1)");
// Draw the Bézier curve instance
instance.draw();
// Schedule the next update cycle with a delay
setTimeout(update, timeout);
}
// Start the animation loop
setTimeout(update, timeout);
Conclusion
Hopefully, this tutorial serves as a solid base for building more complex generative visuals, and encourages you to experiment with and adapt these techniques to your specific needs, whether for games, art installations, or educational tools. Also kindly leave a comment and share any of your projects. We would love to see what you create. Happy creative coding!