Creating Python Addons for Blender 3D
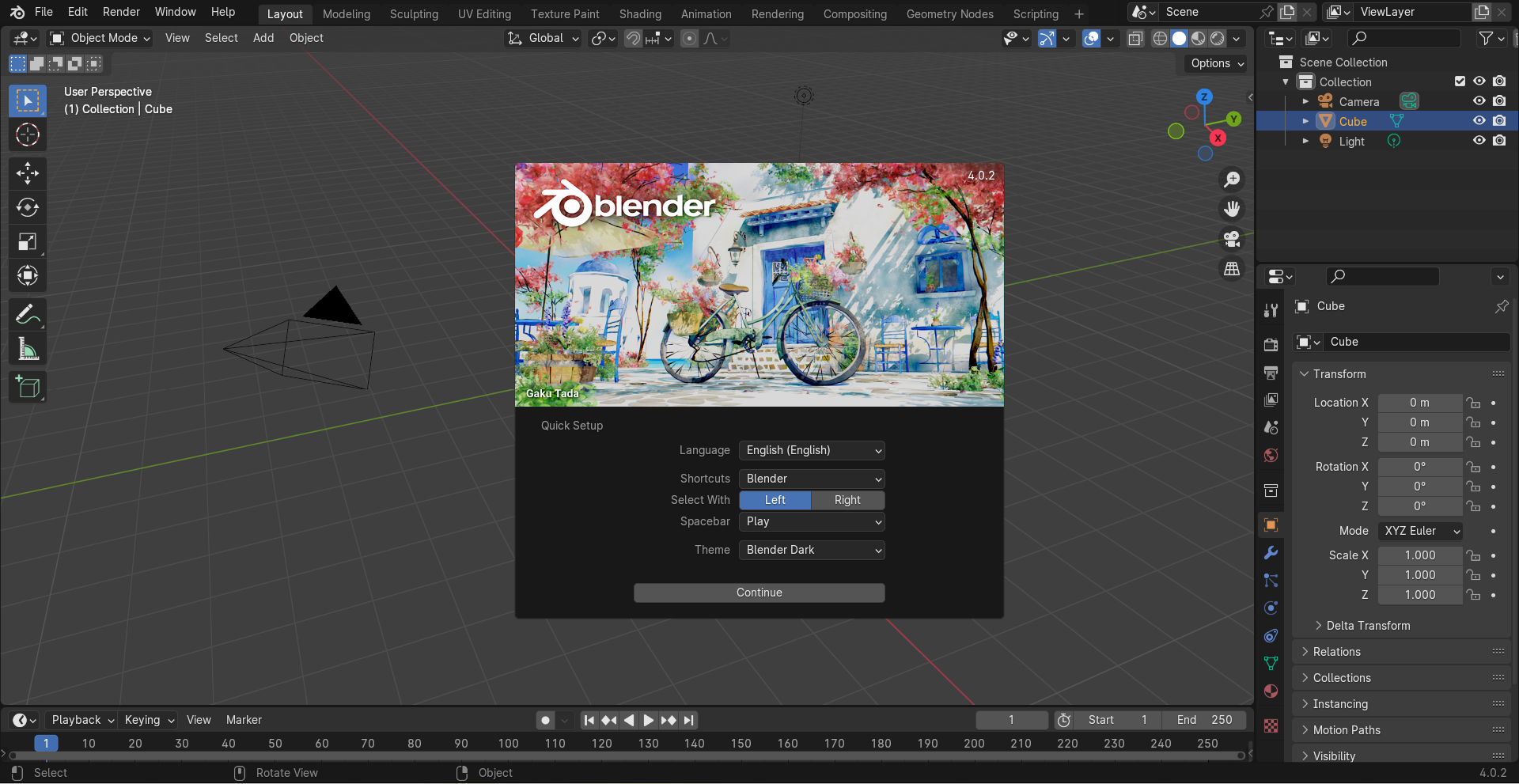
Blender is an open source animation software created in 1994 using the C Programming language. The software is used to create 3D graphics, films and video games. Blender currently has over 1 millions user world wide.
As the number of users has grown, so has the need for the software to be come for versatile. To address this, the developers of the software decided to extend it with Python, giving power to the users to create addons and extensions for software.
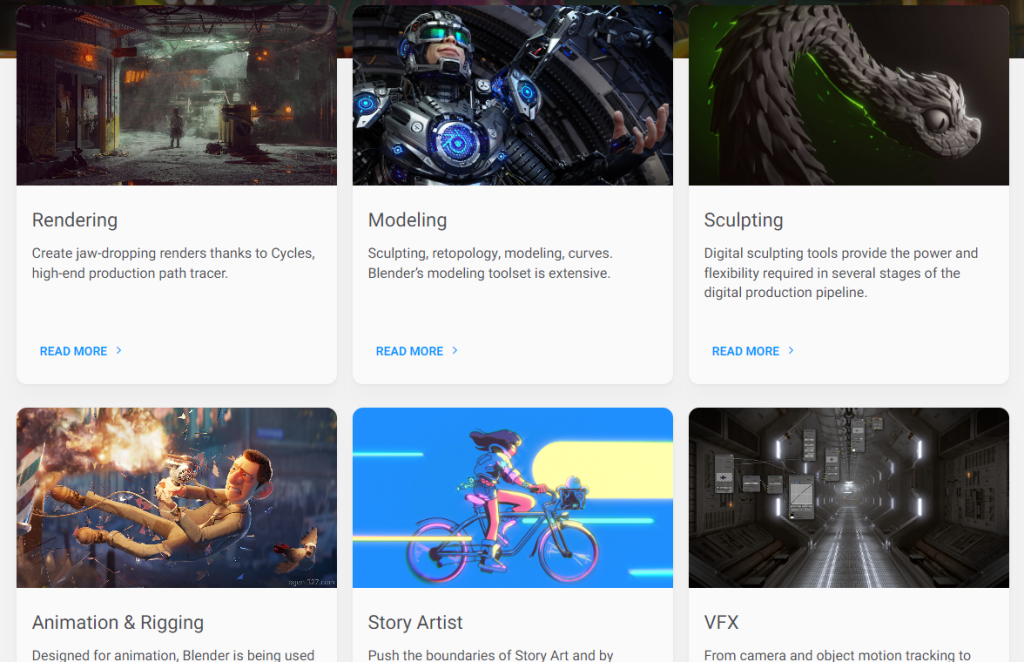
Since Python is one of our favorite programming languages, we decided to create this tutorial on how you can use Python to solve practical problems and cool addons for blender.
Requirements and prerequisites
- Blender Installation: Ensure that you have the latest version of Blender installed on your system. You can download the software from the official Blender website (https://www.blender.org/download/) and follow the installation instructions for your operating system.
- Basic Understanding of Blender Interface: Familiarize yourself with the basic navigation and features of Blender. Here’s a cool to video tutorial to get you started.
- Fundamental Python Knowledge: While this tutorial aims to be beginner-friendly, having a basic understanding of Python programming concepts will be beneficial. Check our tutorials on Python.
Basics of Add-ons in Blender
The basic structure of a Python Blender addon follows a specific format to ensure compatibility and functionality within the Blender environment. Below is an overview of the fundamental components typically found in a Python script that serves as a Blender addon:
- Header Information:
The script begins with comments providing information about the addon, including the name, description, author, version, and Blender version compatibility.
bl_info = {
"name": "MyAddon",
"blender": (2, 80, 0),
"category": "Object",
}
- Import Statements:
Import necessary modules and classes from the Blender Python API (bpy) to access Blender’s functionality.
import bpy
- Operator or Panel Definition:
Define the main operator or panel class that will contain the functionality of the addon. This class typically inherits from bpy.types.Operator or bpy.types.Panel.
class OBJECT_OT_my_operator(bpy.types.Operator):
bl_label = "My Operator"
bl_idname = "object.my_operator"
- Operator or Panel Execution Code:
- Implement the execute method for operators or the draw method for panels. This is where the main functionality of the addon is defined.
def execute(self, context):
# Your operator logic goes here
return {'FINISHED'}
- Registration and Unregistration Functions:
- Define functions to register and unregister the addon. These functions typically include adding the operator or panel to Blender’s internal structures.
def register():
bpy.utils.register_class(OBJECT_OT_my_operator)
def unregister():
bpy.utils.unregister_class(OBJECT_OT_my_operator)
- Addon Testing Code:
- Optionally, include code to test the addon when run as a standalone script. This can be useful during development.
if __name__ == "__main__":
register()
- User Interface Integration:
- If the addon includes a user interface component (such as a panel), ensure that it is registered and added to the appropriate location in Blender’s UI.
Creating your First Blender Addon
For the purpose of this tutorial, we are going to create a simple blender addon to randomly generate cubes in 3D space.
To get started, open up blender and select scripting from the top menu.
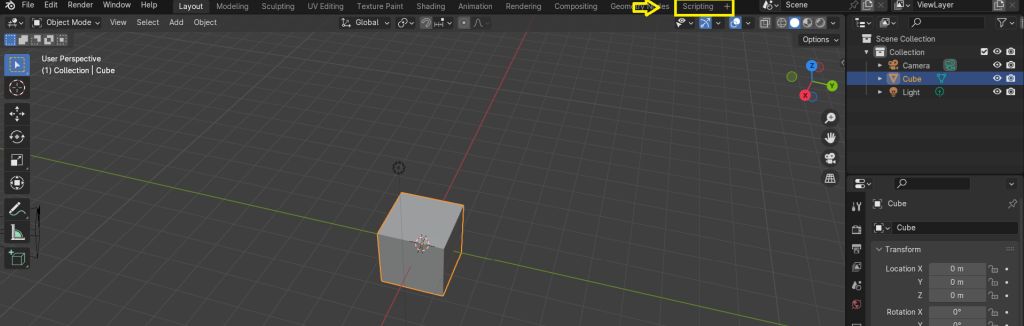
Using the blender text editor create a new python script file called “myaddon.py”.
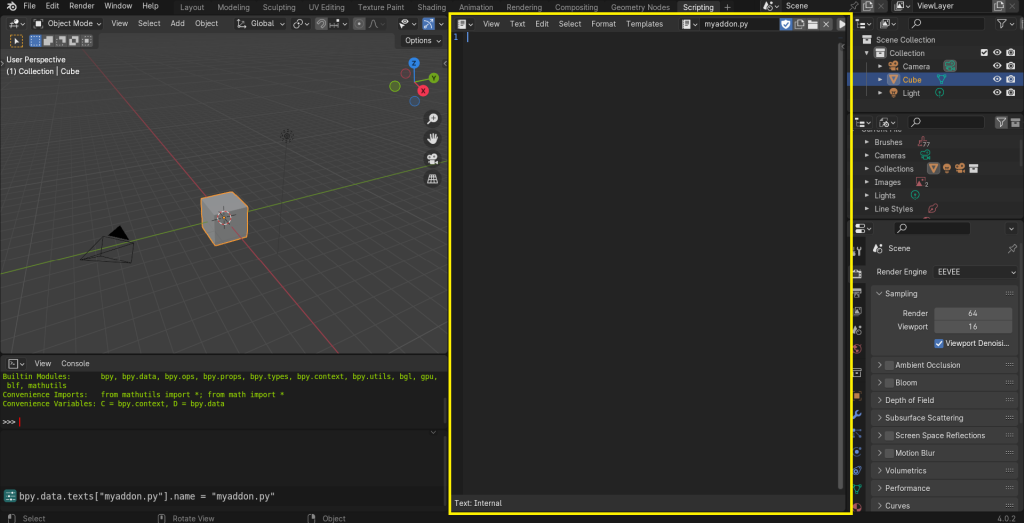
Now, lets write some logic for our addon. Just copy the below code and paste into the text editor, and run it.
import bpy
#lets import the uniform to generate random float numbers
from random import uniform as random
#total of cubes
total_number=100
#lets set the min and max size of the cubes
size=[0.5,1]
#maximum distance of the cubes from the origin
max_distance=10
index=0
#this while loops is run to generate each individual cube.
while(index<total_number):
#lets give the cubes random sizes
cube_size=random(size[0],size[1])
#lets also create random cordinates for the cubes
x=random(-max_distance,max_distance)
y=random(-max_distance,max_distance)
z=random(-max_distance,max_distance)
#lets add the cubes to our scene
bpy.ops.mesh.primitive_cube_add(size=cube_size,enter_editmode=False, align='WORLD', location=(x,y,z))
index+=1
In the scene view, you should see the cubes generated as shown below:
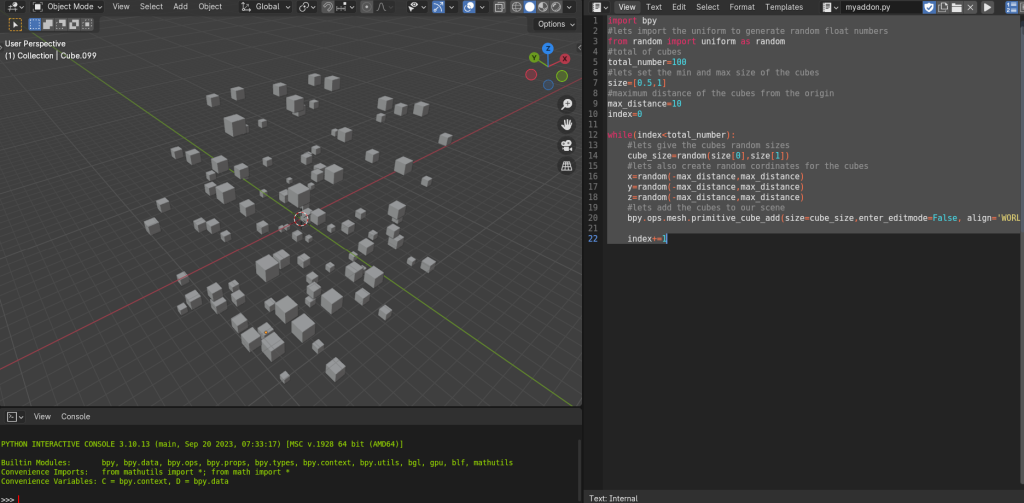
Now lets finalize our addon by writing code to configure and register it within the blender system.
bl_info = {
"name": "MyAddon",
"author": "25scripts",
"version": (1, 0),
"blender": (2, 80, 0),
"location": "View3D > 25scripts",
"description": "Generates Random Cubes",
"warning": "",
"doc_url": "",
"category": "",
}
import bpy
#lets import some classes; the Operator for buttons and Panel for UI panels.
from bpy.types import (Panel,Operator)
#lets import the uniform to generate random float numbers
from random import uniform as random
#lets create the button operator Class
class MyButtonOperator(bpy.types.Operator):
bl_idname = "random.1"
bl_label = "Random Operator"
def execute(self, context):
#total of cubes
total_number=100
#lets set the min and max size of the cubes
size=[0.5,1]
#maximum distance of the cubes from the origin
max_distance=10
#this while loops is run to generate each individual cube.
index=0
while(index<total_number):
#lets give the cubes random sizes
cube_size=random(size[0],size[1])
#lets also create random cordinates for the cubes
x=random(-max_distance,max_distance)
y=random(-max_distance,max_distance)
z=random(-max_distance,max_distance)
#lets add the cubes to our scene
bpy.ops.mesh.primitive_cube_add(size=cube_size,enter_editmode=False, align='WORLD', location=(x,y,z))
index+=1
return {'FINISHED'}
#lets create the UIPanel Class
class MyPanel(bpy.types.Panel):
#lets set the label of the panel
bl_label = "MyAddon"
bl_idname = "OBJECT_PT_myaddon"
bl_space_type = 'VIEW_3D'
bl_region_type = 'UI'
bl_category = "MyAddon: Random Cubes"
def draw(self, context):
layout = self.layout
#lets add the button to the panel.
row = layout.row()
row.operator(MyButtonOperator.bl_idname,text="Generate",icon="CUBE")
#lets import some functions to helps register our addon
from bpy.utils import register_class, unregister_class
#lets set the classes we want to register
classes=[MyButtonOperator,MyPanel]
#now lets register the classes
def register():
for clss in classes:
register_class(clss)
def unregister():
for clss in classes:
unregister_class(clss)
if __name__ == "__main__":
register()
If you press “N” in the scene view, you should be able to see our addon and run it.
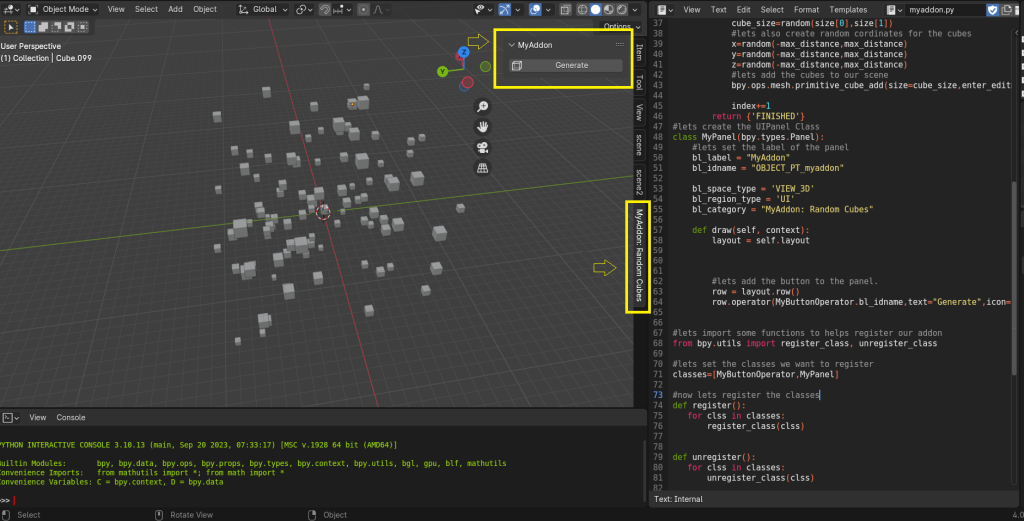
Finally, you can now package your addon for anyone to use. Simply, save the python file to a desired location and then zip it.
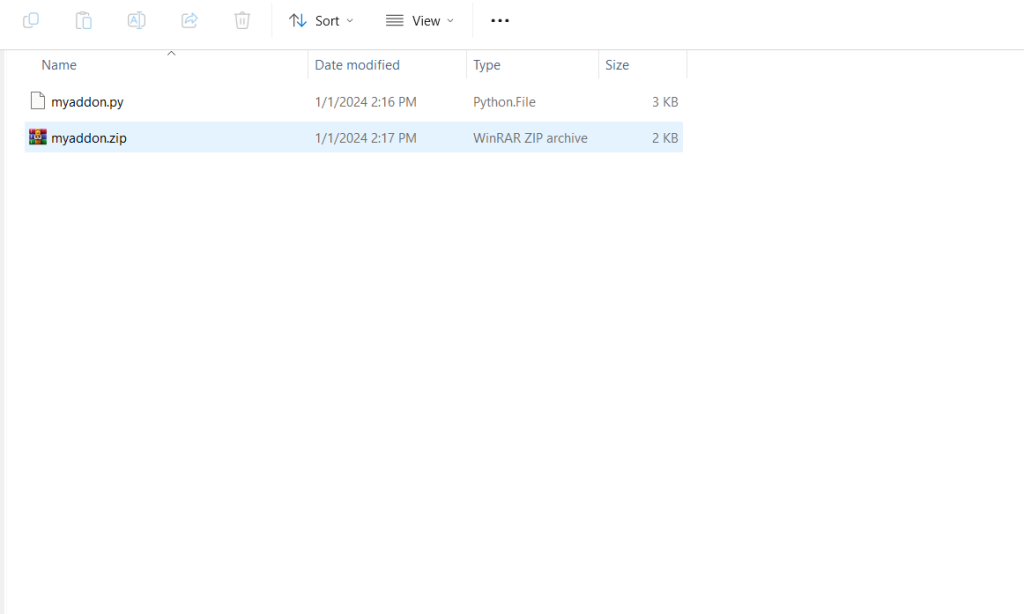
To install the addon, to go the Edit>Preferences>Add-ons and Click on “Install…”. Then select the zip file of your addon. Finally, active your addon and you can start using it.
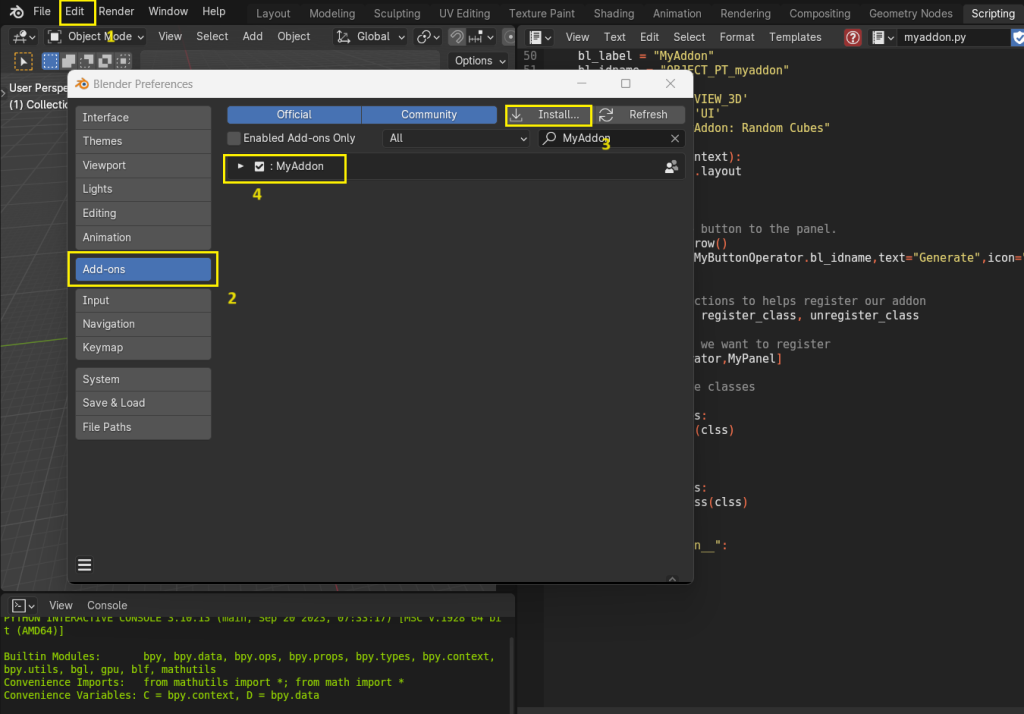